Reference types vs Value types in Swift

In Swift, a reference type is a form of storing data that creates a reference or pointer to a location in memory where the data is stored.
In other words, a reference type shares a single copy of its data.
This means that when you create a reference-type instance, you are not making a new copy of the data but rather a reference to the original one.
Examples of reference types in Swift include classes, functions, and closures.
As an example to understand this more easily:
In this example, Book
is a class with a single property title
.
bookOne
is an instance of Book
with the title "A Game of Thrones (1996)". When bookTwo
is assigned to bookOne
, it now refers to the same instance of Book
.
When the title of bookTwo
is changed to "A Storm of Swords (2000)", it reflects in bookOne
as well. This is because both variables refer to the same instance of Book
.
Please try this code in a playground in Xcode and check for yourself the memory address is same in bookOne
and bookTwo
This is how our bookOne
and bookTwo
are referencing to same object in memory.

On the contrary, a value type is a way of storing data that creates a new copy of the data every time it is assigned to a variable or passed to a function.
In other words, a value type instance keeps a unique copy of its data.
This means that when you create a value type instance, you are creating a completely new copy of the data, not a pointer or a reference to the original data.
Examples of value types in Swift include structs, enums and tuples
Let’s use the same example earlier and change only one word from class(Reference type) to struct(value type):
In this example, Book
is a struct and not class to test out the difference.
If you notice here when the title of bookTwo
is changed to "A Storm of Swords (2000)", it does not not reflect bookOne
anymore. This is because a new instance was created for bookTwo
.
Please try this code in a playground in Xcode and check for yourself that the output for each bookOne
and bookTwo
.
This is how our bookOne
and bookTwo
are referencing to different objects in memory.
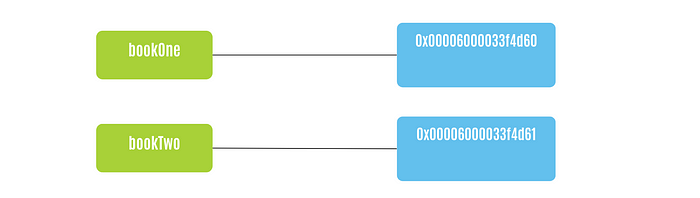
I appreciate you choosing to spend some of your day with me. I hope that my piece was informative and helpful to you.
Farewell, keep growing and learning. See you in the next one. ✌️
Make sure to follow me on Twitter to keep receiving the newest articles: